GenUI Developer Guide
This short tutorial will show you how to extend the GenUI backend framework with new applications and features. We will describe the Python API to create extensions first and then move on to discussing specific feature implementations. This guide assumes you already know a little bit about how the Django web framework works. Skimming through the official Django tutorial should be enough to get you started. We also assume that you have already installed and can run the GenUI backend applications as described in Running from Source.
Extensions
Extensions are nothing special in GenUI. A GenUI extension is nothing more than a basic Django application with a few extras that can make your life a bit easier when integrating the application with the rest of the framework. Here is a high level overview of the GenUI project structure:
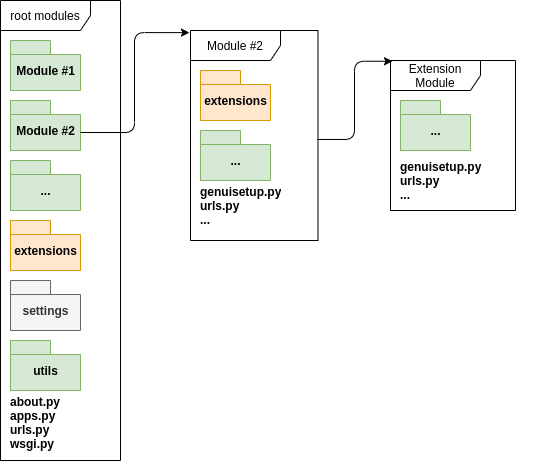
Fig. 1 Overview of the GenUI project structure. The packages (Django applications) on top of the hierarchy are called root packages and they can have any number of subpackages. A special subpackage is the extensions package, which contains applications extending the GenUI framework.
Each root package has its function in the framework and also it’s own submodules and subpackages. A special kind of subpackage is the extensions subpackage. This is a place where applications that are not part of the GenUI Python API are placed. These are packages implementing molecular generators, machine learning algorithms for QSAR and dimensionality reduction, but also data import options and other functionality. In short, extensions are Django applications not necessary for the operation of the GenUI framework itself, but rather applications that only depend on it.
So far you need to place your extensions into these predefined directories. However, soon there will be a feature that will allow you to mark any directory as containing additional extensions.
Todo
Add info about this feature when it is implemented.
Creating an Extension
In order to create an extension, you have to create its root directory first.
Lets say we would like to create an extension that contains a collection of new
QSAR models that we would like to expose in the GenUI REST API. We will name
this extension qsarextra
(see QSAR).
In order for everything to work,
we will have to place it in the genui/qsar/extensions
directory. On Linux we would create this directory on command
line like this from the repository root:
mkdir src/genui/qsar/extensions/qsarextra
Then we can make use of the Django manage.py
command to bootstrap this application for us:
cd src/
python manage.py startapp qsarextra qsar/extensions/qsarextra
This will generate the proper directory structure under genui/qsar/extensions
.
Now we just need to add a few extras to make this package into a GenUI extension.
All it takes is the genuisetup.py
script in genui/qsar/extensions/qsarextra/
.
This module is recognized by the genuisetup
command, which is responsible for
properly initializing extensions. A typical genuisetup.py
looks something like this:
"""
genuisetup.py in src/genui/qsar/extensions/qsarextra/
"""
PARENT = 'genui.qsar'
def setup(*args, **kwargs):
pass
The PARENT
variable is used to denote the root package we are extending
and the setup
method is triggered by the genuisetup
command
and later in this tutorial we will use it to set default permissions for
the data structures we create and to tell the GenUI framework about the
new stuff we implemented.
The last thing we have to do is make our extension discoverable by
including it in the __init__.py
script of the qsar.extensions
package:
"""
__init__.py in src/genui/qsar/extensions/
"""
__all__ = ('qsarextra',)
When you run the genuisetup
command with manage.py
:
# setup the extensions and detect new features
python manage.py genuisetup
you should find a new message in the output informing you about your new extension being successfully setup:
Successful setup for: "genui.qsar.extensions.qsarextra"
Note
If you created new models in your extensions, do not forget to generate migrations and migrate the database when appropriate:
# migrate the database if needed
python manage.py makemigrations
python manage.py migrate
Extending the GenUI Applications
In the following tutorials, you will learn the specific details of implementing extensions for various GenUI applications.